How to make api calls in React.
In this post we will learn how to make api call in react using the fetch api and the popular library axios that is used to make api call to rest api.
An Api stands for Application programming Interface and it refers a set of defined rules that enable different applications to communicate with each other. In this case it enables our react application to communicate with web servers to fetch, post, delete or update specified information.
First you have to create your app.
npx create-react-app myappname
1. Making an api call using the Fetch Api.
According to geekforgeeks The fetch() method in JavaScript is used to request data from a server. The request can be of any type of API that returns the data in JSON or XML. The fetch() method requires one parameter, the URL to request, and returns a promise. In simple terms it can be used to get, post(send information to the server), update or delete information stored on a server and returns it.
We will be using the dummy json users api in all the examples below.
Example 1 of 3: making an api get request using the fetch api in react using useEffect when the page loads.
import { useState, useEffect } from "react";
import "./App.css";
function App() {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchusers = async () => {
let fetchedusers = await fetch("https://dummyjson.com/users");
setUsers(fetchedusers.json());
};
console.log(users);
}, []);
return (
<div className="App">
<h1>Check your console in the browser.</h1>
</div>
);
}
export default App;
Example response after request.
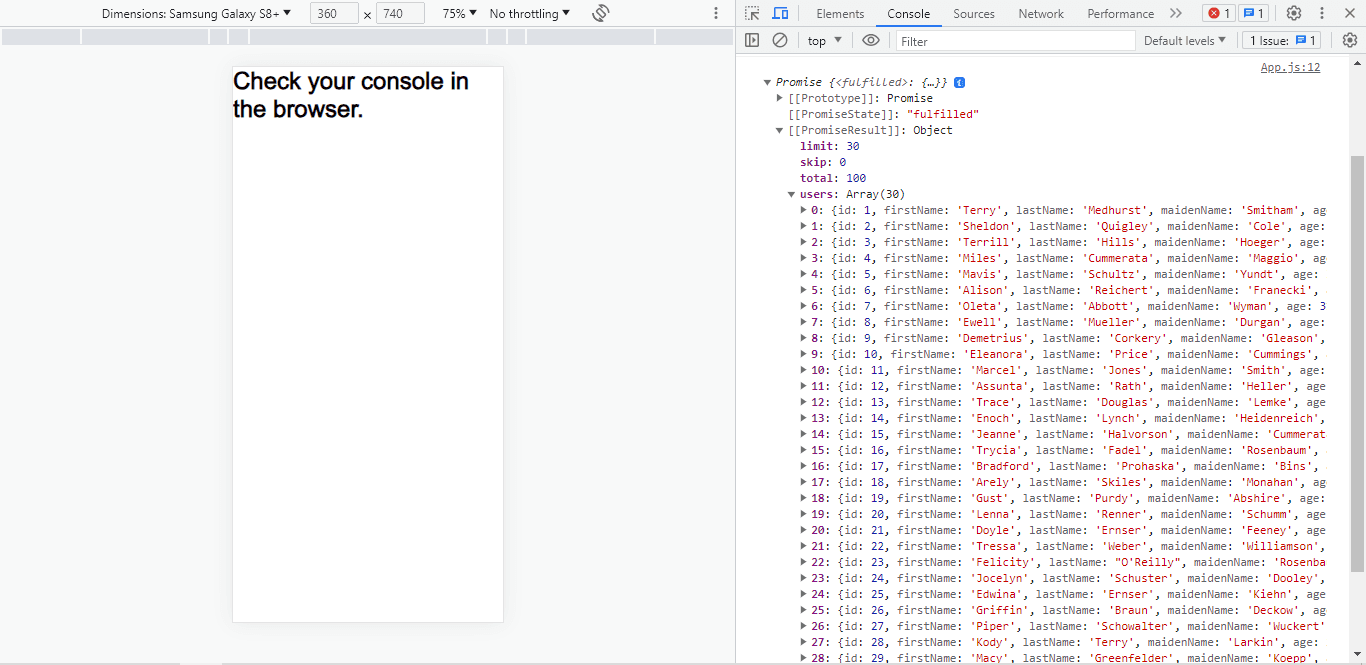
Example 2 of 3: making an api call in react using the fetch api after a button is clicked rather than when the app loads.
import { useState } from "react";
import "./App.css";
function App() {
const [users, setUsers] = useState([]);
const fetchusers = async () => {
const fetchedusers = await fetch("https://dummyjson.com/users");
setUsers(fetchedusers.json());
console.log(users);
};
return (
<div className="App">
<h1>Check your console in the browser.</h1>
{/* users will be fetched after you click this button. */}{" "}
<button onClick={fetchusers}>fetch users</button>
</div>
);
}
export default App;
The response will be the same as above.
Example 3 of 3: making an api post request in react using the fetch api.
Post request this is the adding of information to a web server for example, creating a new user in a social media site.
import { useEffect } from "react";
import "./App.css";
function App() {
useEffect(() => {
fetch("https://dummyjson.com/users/add", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({
firstName: "The",
lastName: "Developer",
age: 20,
gender: "male",
bloodGroup: "0",
height: "7 ft",
/* other user data */
}),
}).then((res) => console.log(res.json()));
}, []);
return (
<div className="App">
<h1>Check your console in the browser.</h1>
</div>
);
}
export default App;
Code explanation.
- The code begins by importing the useEffect hook from the React library.
- The App function is defined, which represents a React component. This component will be rendered and displayed on the web page.
- Inside the App component, the useEffect hook is used. The useEffect hook is a built-in React hook that allows you to perform side effects in functional components. In this case, it is used to send a POST request to the URL "https://dummyjson.com/users/add" when the component is mounted.
- The fetch function is called within the useEffect hook. It sends a POST request to the specified URL with the provided request options. The request includes a JSON payload containing user data such as firstName, lastName, age, gender, bloodGroup, and height.
- After the POST request is completed, the response is logged in the browser console using the console.log function. The res.json() method is called on the response object to parse the response body as JSON.
The empty dependency array ([]) passed as the second argument to useEffect ensures that the effect only runs once, immediately after the component is mounted.
The response.
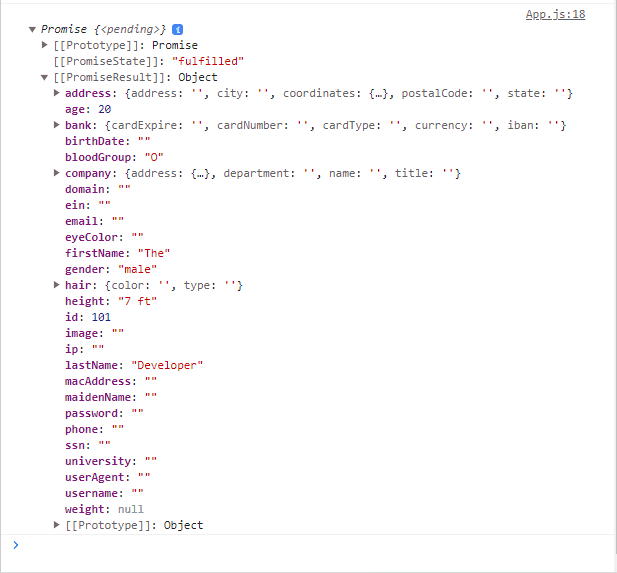
2. Making an api call using the axios library.
Axios is a popular javascript library for making http or api requests to web servers. Axios is used to send asynchronous HTTP requests to REST endpoints, perform necessary operations such as create, read, update and delete(CRUD), and also handle the responses.
To start using axios you have to install it to your project using the command
npm install axios
Example 1 of 2: making a get request to an api using axios library.
import { useState, useEffect } from "react";
import "./App.css";
import axios from "axios";
function App() {
const [users, setUsers] = useState([]);
useEffect(() => {
axios
.get("https://dummyjson.com/users")
.then((res) => setUsers(res.data.users));
console.log(users);
}, []);
return (
<div className="App">
{" "}
<h1>Check your console in the browser.</h1>
</div>
);
}
export default App;
Response.
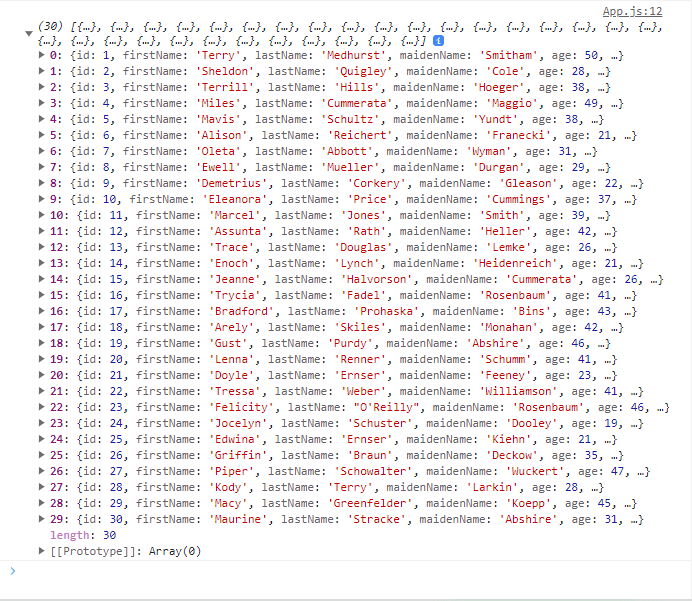
Example 2 of 2: Making an api post request in react using axios.
import { useEffect } from "react";
import "./App.css";
import axios from "axios";
function App() {
useEffect(() => {
const user = {
firstName: "The",
lastName: "Developer",
age: "20",
gender: "male",
bloodGroup: "0",
height: "7 ft",
/* other user data */
};
axios
.post("https://dummyjson.com/users/add", user)
.then((res) => console.log(res.data));
}, []);
return (
<div className="App">
<h1>Check your console in the browser.</h1>
</div>
);
}
export default App;
Response.
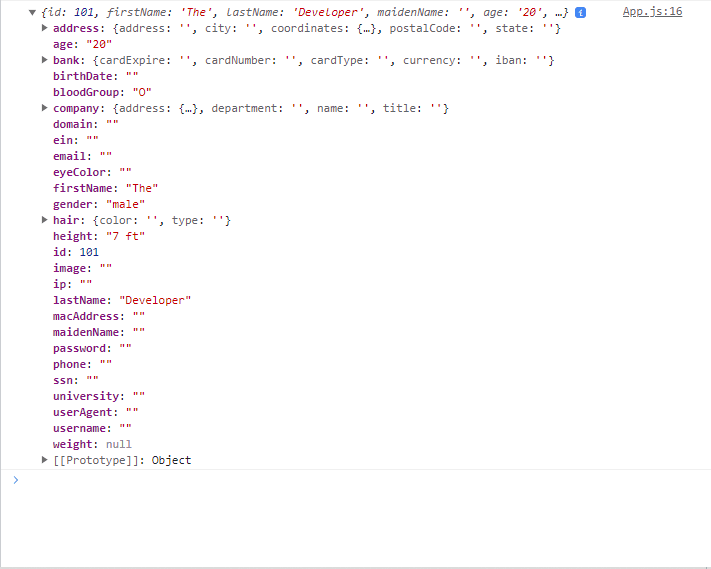
In this tutorial, we have covered the fundamentals of making API calls in React, providing you with a comprehensive understanding of the process. By following our step-by-step instructions, you can confidently integrate external data sources into your React applications. Whether you choose the Fetch API or the Axios library, understanding API calls in React will empower you to create dynamic, data-driven web applications and enhance your development skills. Get ready to unlock the full potential of React by seamlessly integrating APIs into your projects.
Found this article helpful? leave a comment down below.
comments
Add a comment...